Rust - The Modern System Programming Language
A Taste of Rust
Why Rust is Awesome?
Featuring:
- zero-cost abstractions
- move semantics
- guaranteed memory safety
- threads without data races
- trait-based generics
- pattern matching
- type inference
- minimal runtime
- efficient C bindings
Awesomeness #1: Zero-cost abstraction
What you don’t use, you don’t pay for. And further: What you do use,
you couldn’t hand code any better.
[Stroustrup, 1994]
Awesomeness #2: Trait-based generics
- The ONLY notion of interface
- Statically dispatched: C++ templates
- Dynamically dispatched: Java Interface
- Trait bound and inheritance
Play
Awesomeness #3: Memory safety
- A BIG topic in fact
- Static methods: Cyclone, MLKit ... Rust
- Dynamic methods: Garbage collection! Java, Python, C# ....
- Rust has many concepts...
A#3.1: Ownership and Borrowing
Classical problem: Memory leak
- If you own something, you may borrow it out, but you must make
sure that you can get it back
- If you don't want something, you may transfer the ownership, but
don't try to interfere with it after giving out
- If you own something, you must live longer than it
Play
A#3.2: References and Mutability
Classical problem: Concurrent data races
- Similar to C++
- Hardened with ownership control, lifetime and mutability
- Principles:
- one or more references (
&T
) to a resource,
- exactly one mutable reference (
&mut T
).
Play
A#3.3: Lifetimes
Classical problems: Use after free
- I acquire a handle to some kind of resource.
- I lend you a reference to the resource.
- I decide I’m done with the resource, and deallocate it, while you
still have your reference.
- You decide to use the resource.
Play
Awesomeness #4: ADT and Pattern Matching
- Algebraic data-types (functional flavor!)
- Destruct/match: More safe/expressive/elegant then
switch
or
dynamic overload
Play
Awesomeness #5: Type System
- Local type inference
- Generics (Parametric polymorphism)
- Trait-based polymorphism
- Associated types (type families)
- NO support for higher-kinded types yet
Awesomeness #5: Type System
Play
Rust's advantages over C++
-
cargo
ecosystem
- Safety
- Simplicity of design
- Modern language constructs
But Rust is not perfect
- Value-returning flavor error handling
- Lack of OOP
- Too many macros
- Steep learning curve (even for C/C++ hackers)
- Still in fast evolving
- Too many types of pointers, closures, strings ....
Rust in the wild
Misc
- Resource:
- Discussions(in Chinese):
Servo - The Modern Parallel Browser Engine
Motivation
Why Mozilla want to invent a new language to write something to replace
Gecko (in C++)?
- Takes advantage of parallelism at many levels
- Eliminating common sources of bugs and security vulnerabilities
associated with incorrect memory management and data races
How does the effort pay off?
Performance and Benchmark
Performance and Benchmark (Cont.)
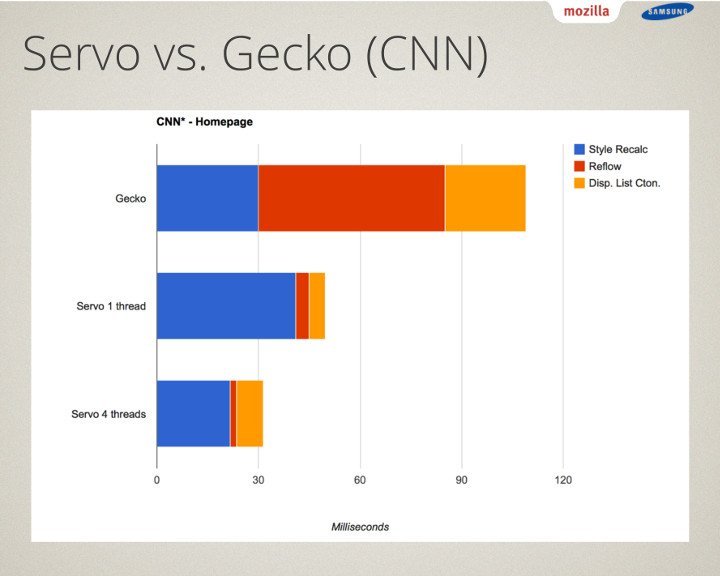
Browser Internals 101
- Layout engine
- JS runtime
- Network/Resource management
- UI
Browser Internals 101 (Cont.)
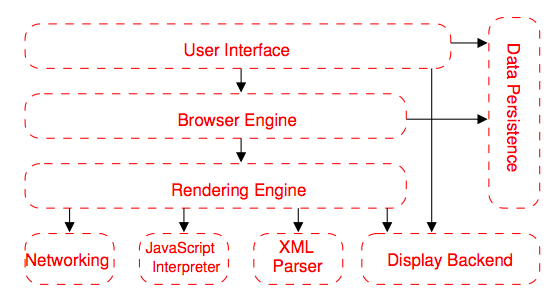
Browser Internals 101 (Cont.)
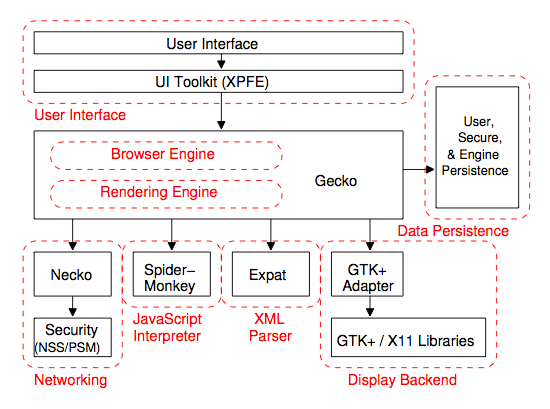
Servo's Architecture
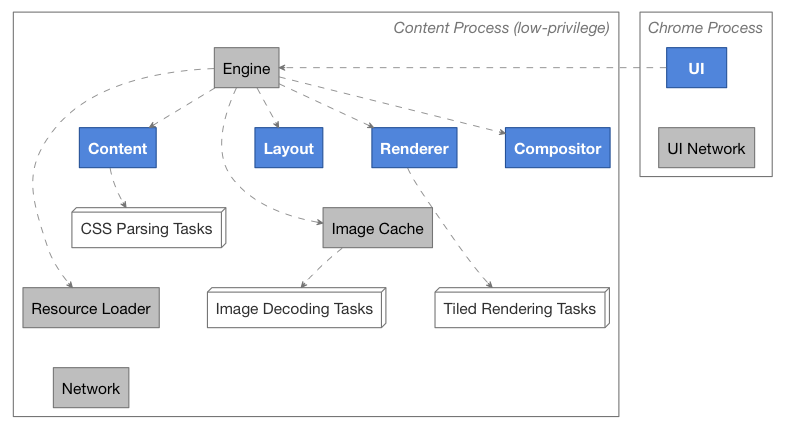
Servo's Architecture (Cont.)
Each constellation manages a pipeline of tasks:
- Script
- Layout
- Renderer
- Compositor
https://github.com/servo/servo/wiki/Design
Servo's Strategies for parallelism and concurrency
- Task-based architecture
- Concurrent rendering
- Tiled rendering
- Layered rendering
- Image decoding
- GC JS concurrent with layout
Personal Experience of Hacking on Servo
- Very mature infra, easy to use tools
- You can learn a lot
- Nice and supportive people in Mozilla
- Very friendly to new contributors
Roadmap
- Ship one Rust component in Firefox Nightly, riding the trains
- Experiment with the uplift of a major piece of Servo into Gecko
- June tech
demo
Servo needs you
Misc
Most Loved PL in 2016 is Rust
https://stackoverflow.com/research/developer-survey-2016
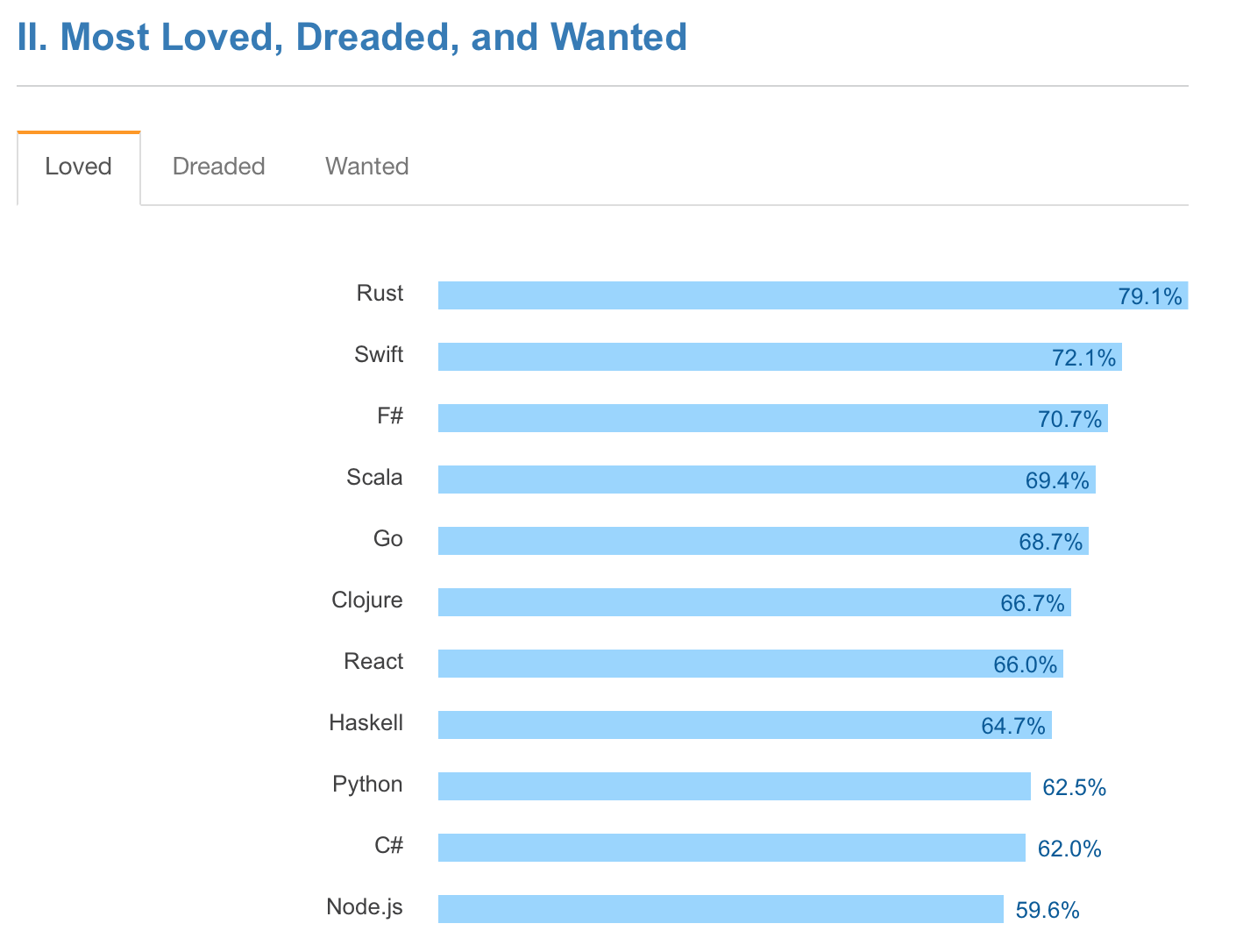
Rust just turns one year old
Rust 1.0 was released at May 15, 2015. Here is a post on the past
year.